Google Sign-In in Flutter: Step-by-Step Guide (2025)
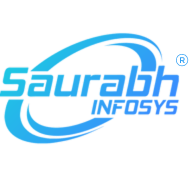
Saurabh Infosys
Flutter Development
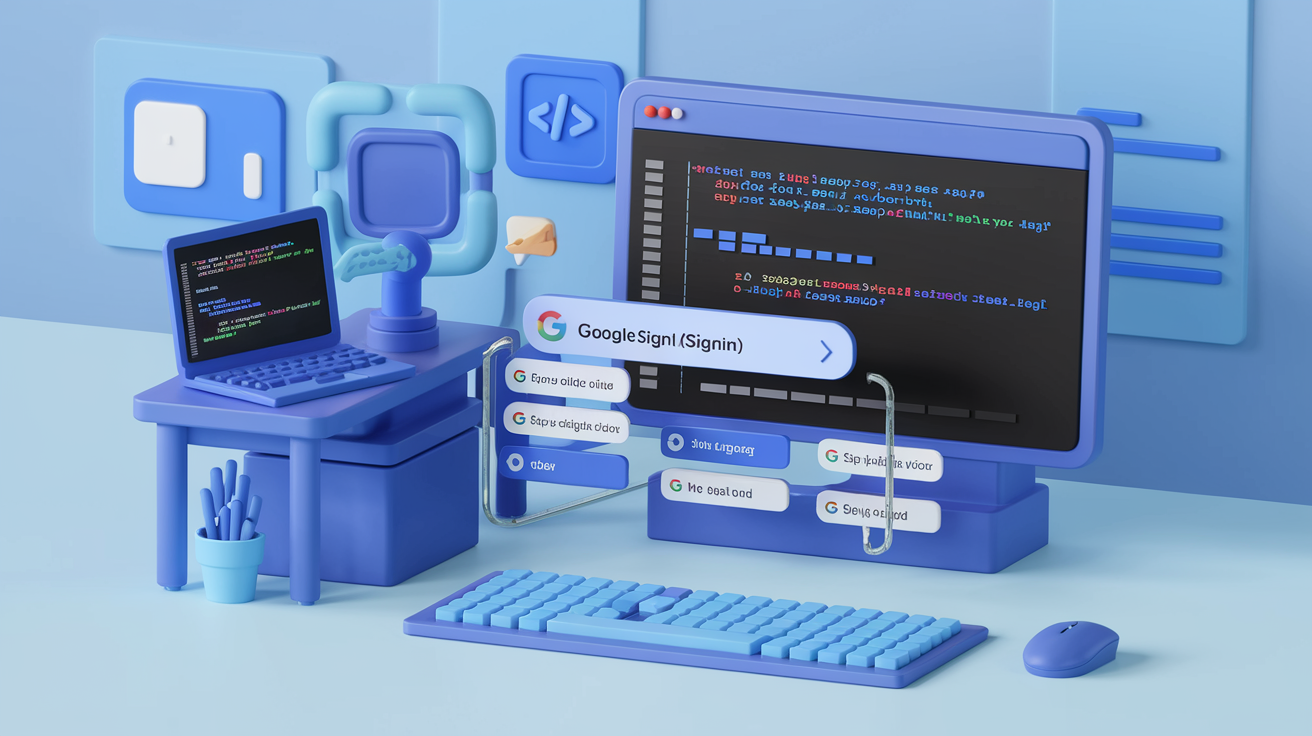
Prerequisites for Google Sign-In in Flutter
Before implementing Google authentication in Flutter, ensure you have:
- A Flutter development environment set up.
- An active Firebase account.
- A new or existing Flutter project ready to integrate with Firebase.
Step 1 – Set Up Your Flutter Project
Install Flutter and set up the development environment.
Create a new Flutter project:
flutter create my_project
cd my_project
Open the project in your preferred code editor (e.g., VS Code or Android Studio).
Step 2 - Configure Firebase for Google Sign-In
- Go to the Firebase Console.
- Create a new Firebase project or select an existing one.
- Add your Android and iOS apps to the Firebase project.
- Download the necessary configuration files:
google-services.json
(Android) → Place inandroid/app/
GoogleService-Info.plist
(iOS) → Place inios/Runner/
- Enable Google Sign-In in Firebase Authentication settings.
Step 3 - Add Dependencies to pubspec.yaml
Add the required packages for Firebase authentication and Google Sign-In:
dependencies:
firebase_core: latest_version
firebase_auth: latest_version
google_sign_in: latest_version
Run the following command to install dependencies:
flutter pub get
Step 4 - Configure Android for Google Sign-In
- Modify
android/build.gradle
andandroid/app/build.gradle
: - Ensure the Google services plugin is applied.
- Update
minSdkVersion
if necessary. - Add:
classpath 'com.google.gms:google-services:latest_version'
AndroidManifest.xml
to include necessary configurations.Step 5 - Configure iOS for Google Sign-In
Open ios/Runner/Info.plist
and add the following:
<array>
<dict>
<key>CFBundleURLSchemes</key>
<array>
<string>YOUR_REVERSED_CLIENT_ID</string>
</array>
</dict>
</array>
Replace YOUR_REVERSED_CLIENT_ID
with the reversed client ID from GoogleService-Info.plist
.
Step 6 – Initialize Firebase
Modify main.dart
to initialize Firebase:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
Step 7 – Implement Google Sign-In in Flutter
Create a new file sign_in_demo.dart
and add the following:
final GoogleSignIn googleSignIn = GoogleSignIn();
final FirebaseAuth auth = FirebaseAuth.instance;
Future signInWithGoogle() async {
final GoogleSignInAccount? googleUser = await googleSignIn.signIn();
final GoogleSignInAuthentication googleAuth = await googleUser!.authentication;
final AuthCredential credential = GoogleAuthProvider.credential(
accessToken: googleAuth.accessToken,
idToken: googleAuth.idToken,
);
final UserCredential userCredential = await auth.signInWithCredential(credential);
return userCredential.user;
}
Add a Google Sign-In button and call signInWithGoogle()
on tap.
Step 8 – Run Your App
Run the app on an emulator or physical device:
flutter run
Test Google Sign-In functionality to ensure proper authentication.
FAQs
Q1: How do I add Google Sign-In to my Flutter app?
A: Follow this guide step by step: Set up Firebase, configure dependencies, update Android/iOS settings, and implement sign-in logic in Dart.
Q2: Why is my Google login in Flutter not working?
A: Common issues include incorrect Firebase configuration, outdated dependencies, missing SHA keys, or incorrect OAuth settings.
Q3: Is Firebase required for Google login in Flutter?
A: Yes, Firebase Authentication simplifies Google Sign-In implementation and manages user authentication securely.
Conclusion
Google Sign-In in Flutter with Firebase provides a seamless authentication experience for your users. By following this guide, you can quickly set up and implement secure user authentication in your app.
Categories
- Mobile Development (1)
- Web Development (1)
Latest Blogs
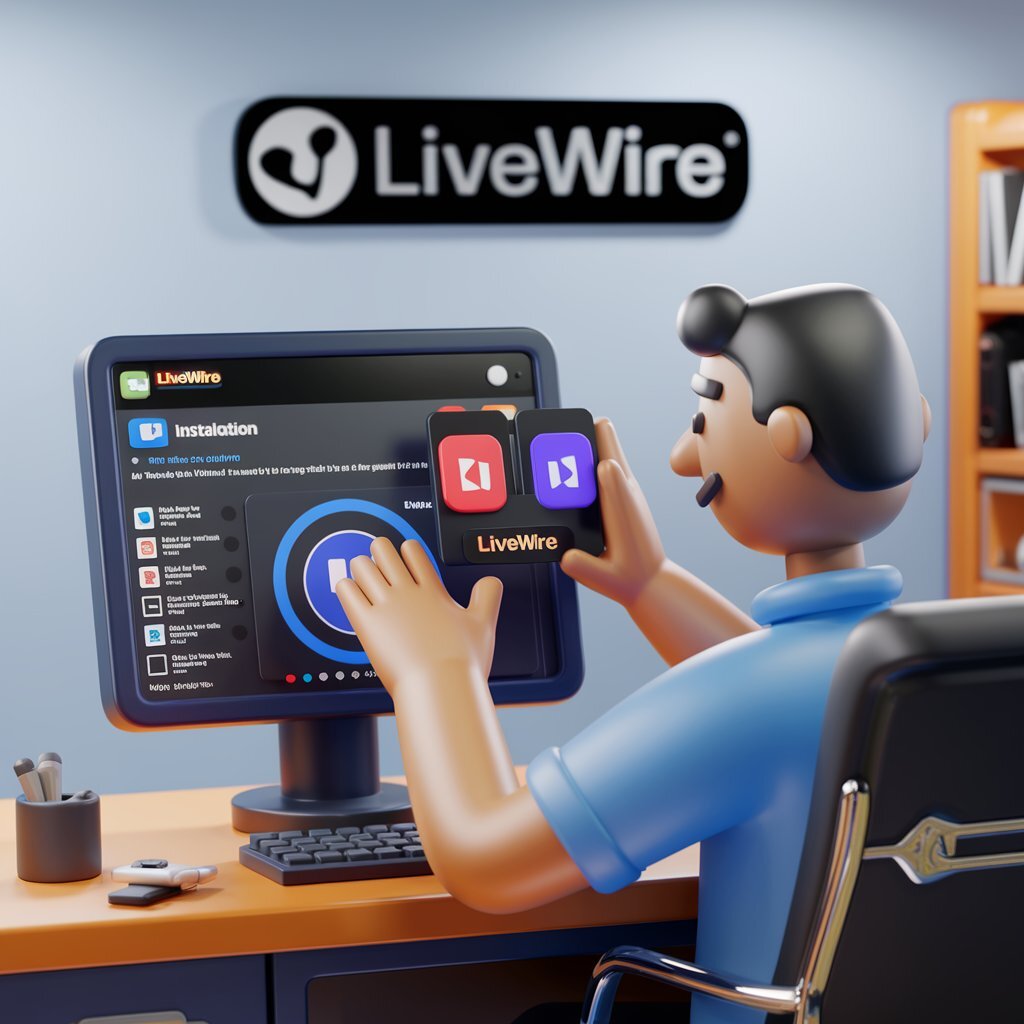
March 18, 2025
Laravel Livewire: Build Dynamic Applications Without JavaScript
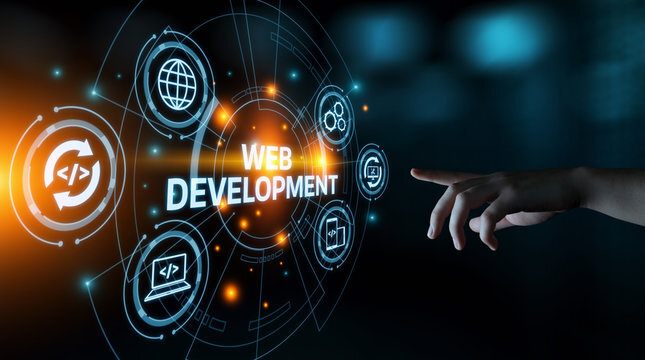
February 3, 2025
Discuss how website speed affects user retention, SEO rankings, and overall performance, and provide tips for improving load times. How to Ensure Your Website is Mobile-Friendly in 2025
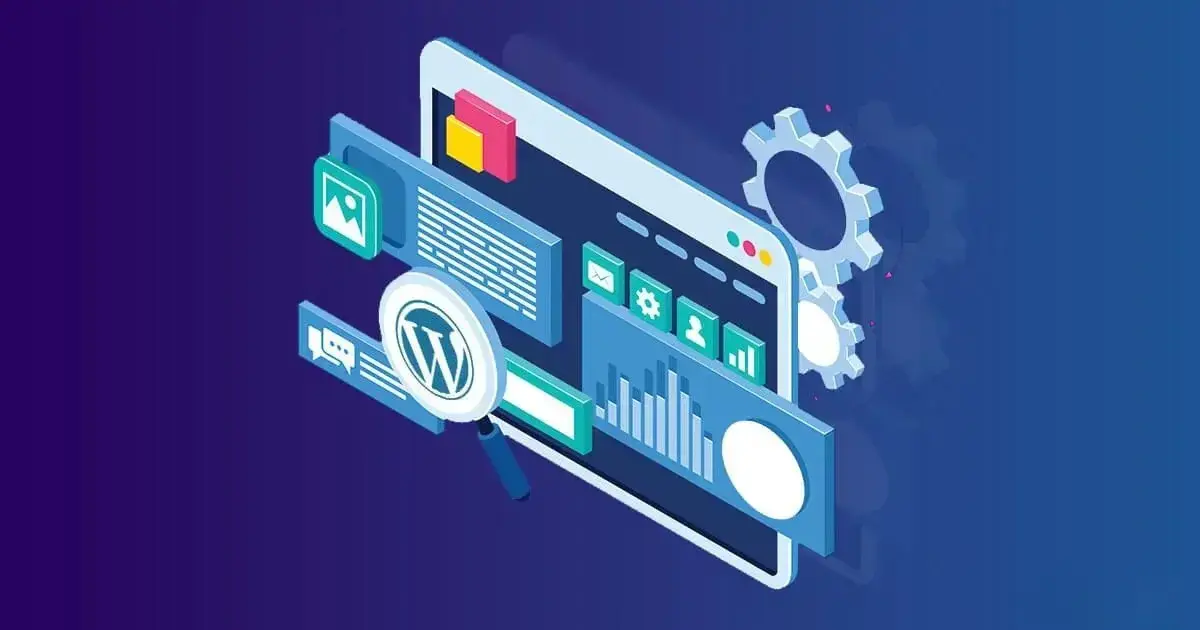
February 3, 2025
Discuss the advantages and limitations of using WordPress for e-commerce websites, including a look at plugins like WooCommerce.
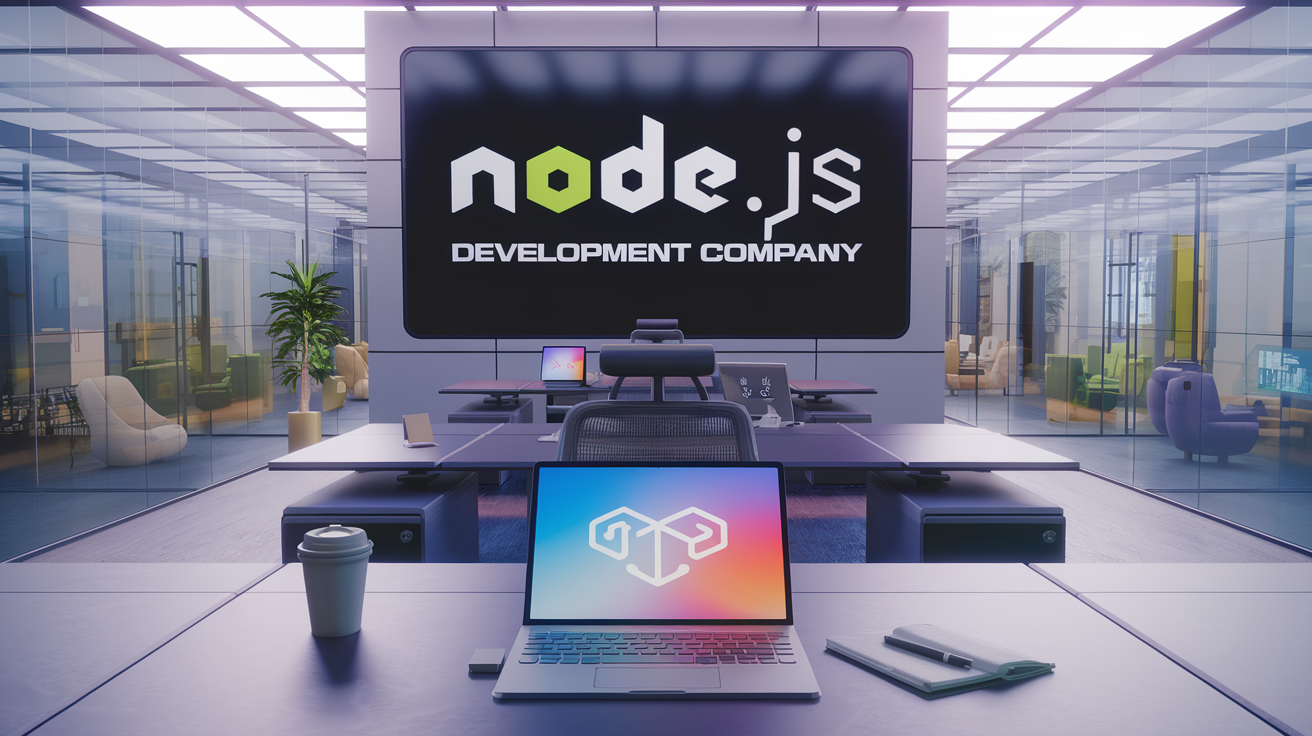
March 29, 2025